Laravel Framework Introduction
Laravel is an open source PHP framework for users working on Web application development. Considered as an alternative to CakePHP and Codelgniter, Laravel uses the Model-View-Controller (MVC) design pattern to facilitate development of Web applications in a quick and easy manner.
DID YOU KNOW?
Laravel is a Web application framework with expressive, elegant syntax. Laravel eases the use of common tasks used in the majority of Web projects, such as authentication, routing, sessions, and caching.
Need for Framework
Hypertext Processor, commonly known as PHP, is an HTML based scripting language that is widely used for Web development.
DID YOU KNOW?
PHP is also one of the first languages learnt by an aspiring Web developer.
PHP was earlier called Personal Home Page and has undergone many version updates to reach its current form. Due to its ease of working, it is one of the most widely supported programming languages, especially by the open source community. The immense support has resulted in the widespread availability of PHP libraries that are available on Internet as open source code.
Although there are a variety of libraries available, it is important to meet the standards and guidelines defined for writing Web application codes, which is a time-consuming process. It also takes effort to find out accurate information on the Internet, install dependencies, configure each library, and include them in different files. This is where frameworks are useful.
A framework is a basic templating structure that is used to develop, test, and deploy applications rapidly and efficiently. Using framework helps a developer focus on the actual problem and application logic, and provides tools to perform tasks easily. It is also used for following purposes:
- To provide abstraction
- To handles repetitive and menial tasks
- To arranges things in a logical structure
The Laravel framework reuses and assembles existing components, dependencies, and libraries to automate time-consuming tasks. This helps a Web developer to build Web applications in a structured manner.
Features of Laravel Framework
Laravel framework includes a consistent API and includes several components to perform common tasks such as database interactions with ease.
Convention over Configuration
Laravel has many conventions. If these conventions are properly followed, it can predict the dependencies and automate the configuration related tasks. The Convention over Configuration feature of Laravel reduces the time and effort required to set up and configure services. Such environments are suitable for Rapid Application Development.
One simple example of Convention over Configuration can be seen in the process of using models to retrieve records stored in a table. If a Model is created with the name Employee, then it will implicitly try to retrieve records from a table name employees, which is the plural snake case name of Employee. If the table name is not the plural snake case name of the Model, then the table name would have to be explicitly defined in the code of the Model.
DID YOU KNOW?
Rapid Application Development is a development model. It includes rapid prototyping. Developers use this model to make multiple iterations and updates to software without a development schedule from scratch.
MVC Architecture
Since, Laravel is based on the Model-View-Controller (MVC) architecture, the programming logic is divided into the following:
Models – Models define the way data is visualized and stored. Models are objects that represent resources utilized in an application. Usually, they are used to handle records in a database. Hence, models can be used to represent different entities, such as an employee or a user or an item. Models are objects that are instances of the Eloquent’s base Model class. This object can be efficiently used to hold records from a database table and perform operations on it. The Eloquent ORM programming techniques allow models to interact more with records in a database.
Views – Views define the way Web pages appear. They are responsible for generating Web pages returned as responses with the help of a controller.
They can be written as standard PHP files or can be dynamically built using the Blade Templating language, which gives a modular approach of creating them. Blade allows complex views to be created from simpler layouts.
Controllers – Controllers define the requisite responses for different requests. In simpler terms, controllers take a request and then, depending on the request generate appropriate responses. Similar to a server, controllers are where the actual processing of requests takes place, such as handling form submissions and interacting with the databases.
All of these have powerful classes that define a lot of methods. These methods do most of the work and provide abstraction from the underlying code. This allows a developer to focus more on what logic should be implemented than how it should be implemented.
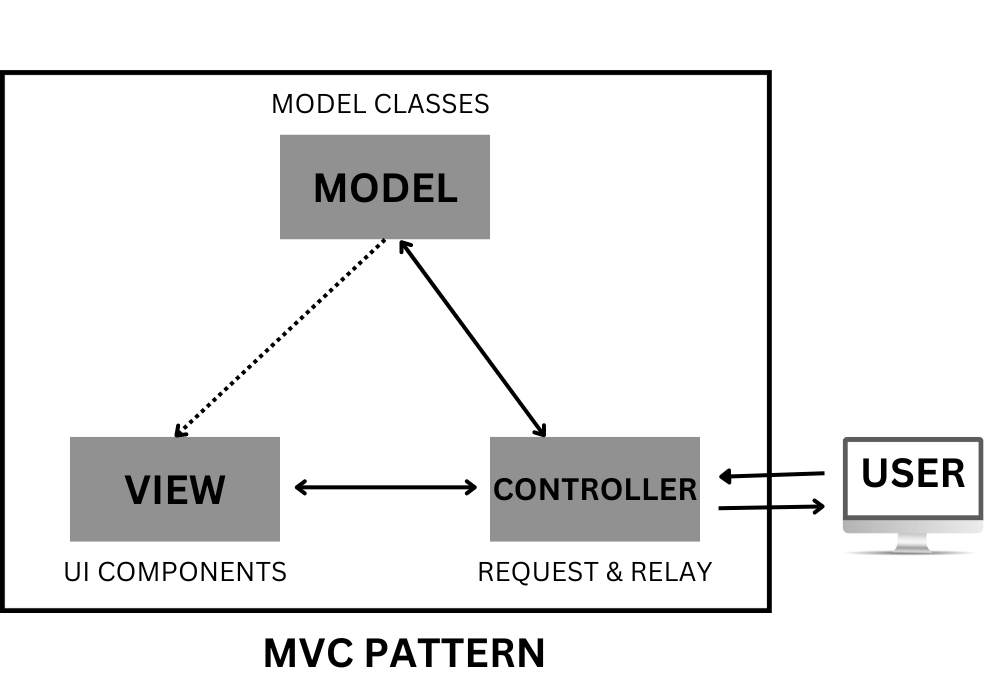
Helper Functions
There are many commonly used functions in Web application development that may require a developer to import different libraries. Laravel includes a variety of global PHP functions called Helpers that help in reducing time and efforts of a developer by taking care of commonly used operations.
Laravel itself utilizes these helper functions in its classes, functions, and even in configurations. This classifies these helpers in the following categories:
- Arrays and Objects: These helpers can be used to perform operations on Arrays and objects. Some operations include sorting values (Arr:sort()), getting the value of the first (head()) or last element (last)), and setting values at a position.(Arr:set()).
- Paths: These helpers store the paths of commonly used locations such as the path of the resource directory (resource_path()), path of the app directory (app_path()), and path of the configuration directory (config_path()).
- Strings: These helpers can be used to perform operations on strings such as finding if it contains another substring(Stricontains()), and transforming the string into camel case(Stracamel()).
- URLs: These helpers can be used to sanitize or generate URLs, such as sanitizing a url into a secure url.
- Miscellaneous: The operations that do not fit any categories are present in miscellaneous, such as finding values of environment variables (env()), finding current date (today()), and redirecting the user to another url (redirect()).
Extensive Library
Laravel is a framework that includes a lot of popular and commonly used libraries. These libraries would have to be manually installed and configured when creating a Web Application without Laravel. Authentication is one such example, which can be easily used to authenticate users while taking care of security issues such as handling attacks that includes SQL Injection, Authentication bypass, and Cross-site Request forgery, encryption, and logging.
Code Snippet
composer require <library-name>
Exploring the Laravel Framework Directory Structure
To explore the directory structure, first create a Laravel application. This can be done by executing the command as shown in Code Snippet 3 in the Homestead directory through ssh.
Code Snippet
laravel new laravelproject
This creates a new directory laravelProject which will contain all the files and directories required for the Laravel Framework
cd laravelproject
is
Directory Structure
The directory structure in Laravel is divided into the following logical parts:
- The app directory: Contains the core code that runs the Laravel application. Most classes are stored in this directory and its subdirectories.
- The bootstrap directory: Includes the code that collates all files spread throughout the directories and run the application.
- The config directory: Includes files related to configuration of different components.
- The database directory: Contains database migrations, seeds, model factories, and the SQLite database that will be used in subsequent sessions.
- The public directory: Includes files that must be exposed to the Internet. Thus, the index.php file calls the app.php file in the bootstrap directory to start the application whenever it receives a request. All HTML, ess, and Image files are also stored in this directory.
- The resources directory: Includes the Views that will be used to display the final Web page as well as the un-compiled assets related to javascript and CSS.
- The routes directory: Provides the route definition for the application that will match the request with its response.
- The storage directory: Includes data generated by the Laravel framework for storage and cache.
- The tests directory: Contains th e test specifications for automated testing.
- The vendor directory: Contains information on third party dependencies imported by the composer dependency manager.
Summary
- Laravel is an open source PHP framework for users working on Web application development.
- A framework is a basic templating structure that is used to develop, test, deploy applications rapidly and efficiently.
- The Laravel framework reuses and assembles existing components, dependencies, and libraries to automate time-consuming tasks.
- Laravel includes a consistent API.
- Laravel has many conventions, if followed, can predict the dependencies and automate the configuration related tasks.
- Laravel is based on the Model-View-Controller (MVC) architecture.
- Laravel utilizes helper functions in its classes, functions, and even configurations.
Leave a Reply